Order Now
- Home
- About Us
-
Services
-
Assignment Writing
-
Academic Writing Services
- Urgent Assignment Help
- Writing Assignment for University
- College Assignment Help
- SPSS Assignment Help
- HND Assignment Help
- Architecture Assignment Help
- Total Assignment Help
- All Assignment Help
- My Assignment Help
- Student Assignment Help
- Instant Assignment Help
- Cheap Assignment Help
- Global Assignment Help
- Write My Assignment
- Do My Assignment
- Solve My Assignment
- Make My Assignment
- Pay for Assignment Help
-
Management
- Financial Management Assignment Help
- Business Management Assignment Help
- Management Assignment Help
- Project Management Assignment Help
- Supply Chain Management Assignment Help
- Operations Management Assignment Help
- Risk Management Assignment Help
- Strategic Management Assignment Help
- Logistics Management Assignment Help
- Global Business Strategy Assignment Help
- Consumer Behavior Assignment Help
- MBA Assignment Help
- Portfolio Management Assignment Help
- Change Management Assignment Help
- Hospitality Management Assignment Help
- Healthcare Management Assignment Help
- Investment Management Assignment Help
- Market Analysis Assignment Help
- Corporate Strategy Assignment Help
- Conflict Management Assignment Help
- Marketing Management Assignment Help
- Strategic Marketing Assignment Help
- CRM Assignment Help
- Marketing Research Assignment Help
- Human Resource Assignment Help
- Business Assignment Help
- Business Development Assignment Help
- Business Statistics Assignment Help
- Business Ethics Assignment Help
- 4p of Marketing Assignment Help
- Pricing Strategy Assignment Help
- Nursing
-
Finance
- Finance Assignment Help
- Do My Finance Assignment For Me
- Financial Accounting Assignment Help
- Behavioral Finance Assignment Help
- Finance Planning Assignment Help
- Personal Finance Assignment Help
- Financial Services Assignment Help
- Forex Assignment Help
- Financial Statement Analysis Assignment Help
- Capital Budgeting Assignment Help
- Financial Reporting Assignment Help
- International Finance Assignment Help
- Business Finance Assignment Help
- Corporate Finance Assignment Help
-
Accounting
- Accounting Assignment Help
- Managerial Accounting Assignment Help
- Taxation Accounting Assignment Help
- Perdisco Assignment Help
- Solve My Accounting Paper
- Business Accounting Assignment Help
- Cost Accounting Assignment Help
- Taxation Assignment Help
- Activity Based Accounting Assignment Help
- Tax Accounting Assignment Help
- Financial Accounting Theory Assignment Help
-
Computer Science and IT
- Robotics Assignment Help
- Operating System Assignment Help
- Data mining Assignment Help
- Computer Network Assignment Help
- Database Assignment Help
- IT Management Assignment Help
- Network Topology Assignment Help
- Data Structure Assignment Help
- Business Intelligence Assignment Help
- Data Flow Diagram Assignment Help
- UML Diagram Assignment Help
- R Studio Assignment Help
-
Law
- Law Assignment Help
- Business Law Assignment Help
- Contract Law Assignment Help
- Tort Law Assignment Help
- Social Media Law Assignment Help
- Criminal Law Assignment Help
- Employment Law Assignment Help
- Taxation Law Assignment Help
- Commercial Law Assignment Help
- Constitutional Law Assignment Help
- Corporate Governance Law Assignment Help
- Environmental Law Assignment Help
- Criminology Assignment Help
- Company Law Assignment Help
- Human Rights Law Assignment Help
- Evidence Law Assignment Help
- Administrative Law Assignment Help
- Enterprise Law Assignment Help
- Migration Law Assignment Help
- Communication Law Assignment Help
- Law and Ethics Assignment Help
- Consumer Law Assignment Help
- Science
- Biology
- Engineering
-
Humanities
- Humanities Assignment Help
- Sociology Assignment Help
- Philosophy Assignment Help
- English Assignment Help
- Geography Assignment Help
- Agroecology Assignment Help
- Psychology Assignment Help
- Social Science Assignment Help
- Public Relations Assignment Help
- Political Science Assignment Help
- Mass Communication Assignment Help
- History Assignment Help
- Cookery Assignment Help
- Auditing
- Mathematics
-
Economics
- Economics Assignment Help
- Managerial Economics Assignment Help
- Econometrics Assignment Help
- Microeconomics Assignment Help
- Business Economics Assignment Help
- Marketing Plan Assignment Help
- Demand Supply Assignment Help
- Comparative Analysis Assignment Help
- Health Economics Assignment Help
- Macroeconomics Assignment Help
- Political Economics Assignment Help
- International Economics Assignments Help
-
Academic Writing Services
-
Essay Writing
- Essay Help
- Essay Writing Help
- Essay Help Online
- Online Custom Essay Help
- Descriptive Essay Help
- Help With MBA Essays
- Essay Writing Service
- Essay Writer For Australia
- Essay Outline Help
- illustration Essay Help
- Response Essay Writing Help
- Professional Essay Writers
- Custom Essay Help
- English Essay Writing Help
- Essay Homework Help
- Literature Essay Help
- Scholarship Essay Help
- Research Essay Help
- History Essay Help
- MBA Essay Help
- Plagiarism Free Essays
- Writing Essay Papers
- Write My Essay Help
- Need Help Writing Essay
- Help Writing Scholarship Essay
- Help Writing a Narrative Essay
- Best Essay Writing Service Canada
-
Dissertation
- Biology Dissertation Help
- Academic Dissertation Help
- Nursing Dissertation Help
- Dissertation Help Online
- MATLAB Dissertation Help
- Doctoral Dissertation Help
- Geography Dissertation Help
- Architecture Dissertation Help
- Statistics Dissertation Help
- Sociology Dissertation Help
- English Dissertation Help
- Law Dissertation Help
- Dissertation Proofreading Services
- Cheap Dissertation Help
- Dissertation Writing Help
- Marketing Dissertation Help
- Programming
-
Case Study
- Write Case Study For Me
- Business Law Case Study Help
- Civil Law Case Study Help
- Marketing Case Study Help
- Nursing Case Study Help
- Case Study Writing Services
- History Case Study help
- Amazon Case Study Help
- Apple Case Study Help
- Case Study Assignment Help
- ZARA Case Study Assignment Help
- IKEA Case Study Assignment Help
- Zappos Case Study Assignment Help
- Tesla Case Study Assignment Help
- Flipkart Case Study Assignment Help
- Contract Law Case Study Assignments Help
- Business Ethics Case Study Assignment Help
- Nike SWOT Analysis Case Study Assignment Help
- Coursework
- Thesis Writing
- CDR
- Research
-
Assignment Writing
-
Resources
- Referencing Guidelines
-
Universities
-
Australia
- Asia Pacific International College Assignment Help
- Macquarie University Assignment Help
- Rhodes College Assignment Help
- APIC University Assignment Help
- Torrens University Assignment Help
- Kaplan University Assignment Help
- Holmes University Assignment Help
- Griffith University Assignment Help
- VIT University Assignment Help
- CQ University Assignment Help
-
Australia
- Experts
- Free Sample
- Testimonial
COIT20256 Data Structure and Algorithms Assignment Sample
Objectives
In this assignment you will develop an application which uses inheritance, polymorphism, interfaces and exception handling. This assignment must be implemented:
1. incrementally according to the phases specified in this documentation. If you do not submit a complete working and tested phase you may not be awarded marks.
2. according to the design given in these specifications. Make sure your assignment corresponds to the class diagram and description of the methods and classes given in this document. You must use the interface and inheritance relationships specified in this document. You must implement all the public methods shown. Do not change the signature for the methods shown in the class diagram. Do not introduce additional public methods unless you have discussed this with the unit coordinator - they should not be required. You may introduce your own private methods if you need them. Make sure your trace statements display the data according to the format shown in the example trace statements. This will be important for markers checking your work.
3. Using the versions of the software and development tools specified in the unit profile and on the unit website.
Phase 1
Description
In phase 1, you are to simulate customer groups of two people arriving every two time units for 20 time units. The only event types that will be processed in this initial phase are Arrival Events. There will be no limit to the number of customers in the shop in this phase. However, this phase for assignment help will require you to set up most of the framework needed to develop the rest of the simulation. At the end of the phase 1 simulation you are to display all the customer groups currently in the shop and all the customer groups that have ever arrived at the shop (this will be a history/log of customers that could be used for future analysis). Given that leave events will not be implemented in this phase, the customer groups in the shop will correspond to the customer groups in the log/history.
Phase 2
In phase 2 we will now have the groups get their coffee order after they arrive. This will be achieved by introducing a new Event type (an OrderEvent) to be scheduled and processed. To do this we have to:
1. Add a serveOrder() method to the ShopModel class.
This method is to print out a message to indicate the time that the group involved has been given their coffee (i.e. print out the following message:
“t = time: Order served for Group groupId”
where the words in italics and bold are to be the actual group id and time. The signature for the serveOrder () method is: public void serveOrder(int time, CustomerGroup g)
2. Add an OrderEvent class
The OrderEvent extends the abstract Event class and must implement its own process() method.
The OrderEvent’s process() method must use the shop model’s serveOrder() method to get a coffee order filled for the group (i.e. print out the message). (In a later phase the process model will also schedule the “LeaveEvent” for the group.)
The OrderEvent must also have an instance variable that is a reference to the customer group associated with the order so that it knows which group is being served their coffee.
This means the signature for the OrderEvent’s constructor will be: public OrderEvent(int time, CustomerGroup group)
3. Have the ArrivalEvent’s process() method schedule the new OrderEvent one time unit after the group arrives
4. What should be output by the program at this point? Run and test it.
Phase 3
In phase 3 we will have people leave after they have finished their coffee. To achieve this, we need to:
1. Implement a leave() method in the shop model, which is to:
print a message when a group leaves the shop as follows:
“t = time: Group groupId leaves”
where the words in italics and bold are to be the actual group id and time remove the group from the ArrayList and decrement the number of groups (numGroups) in the shop.
2. Introduce a LeaveEvent class. The LeaveEvent class must:
Extend the abstract Event class
Have a reference to the “customer group” that is about to leave
Implement its own process() method which is to invoke the shop model’s leave method.
3. Have the OrderEvent schedule a LeaveEvent for the group 10 time units after they receive their coffee.
4. What should be output by the program at this point? Run and test it.
Phase 4
Now there are restrictions on the number of customers allowed in the shop. This will correspond to the number of seats made available in the shop. *** For the purpose of testing and to allow the markers to check your output, make the number of seats in the shop 8.
Extend the ShopModel class as follows:
1. Add a ShopModel constructor that has one parameter that is to be passed the number of seats available in the shop when the ShopModel object is created.
2. Include an instance variable in the ShopModel class that records the number of seats available.
The number of seats available is to be passed into the ShopModel constructor. (The UML diagram in Fig 2 now includes this constructor.)
3. When customers arrive in the shop, if there are enough seats available for all the people in the group, then they can enter the shop and get coffee (i.e. schedule an OrderEvent) as normal.
4. If there are sufficient seats to seat all members of the group, print a message as follows:
“t = time : Group groupId (number in group) seated”
where the words in italics and bold are to be the actual group id and time.
In that case, if new customers are seated, the number of seats available in the shop should be decremented. If there are insufficient seats for the group, then these customers cannot enter the shop. In that case, print a message saying: “t = time : Group groupId leaves as there are insufficient seats for the group”
where the words in italics and bold are to be the actual group id and time.
Assume that if there are insufficient seats the group just “goes away” (i.e. does not get added to the ArrayList of Customer Groups in the shop) and are considered lost business. They should still be recorded in the log/history.
Keep track of how many customers are lost business during the simulation and print that information as part of the statistics at the end of the simulation. In both cases (seated or not seated), the process method should schedule the next arrival.
5. Don’t forget to increase the number of seats available when a customer group leaves the shop.
6. At the end of the simulation, print the following statistics:
How many customers were served (requires an instance variable to track this)
How many customers were lost business (requires an instance variable to track this)
Display the groups still in the shop at the end of the simulation
Display the log/history of customer groups that “arrived” in the simulation.
Phase 6
In a simulation you do not normally use the same values for arrival times, times to be served etc. As explained earlier, in practice these values are normally selected from an appropriate statistical distribution. To model this type of variability we are going to use a simple random number generator in a second version of your program. Copy your version 1 program to Assignment1V2. Now add a random number generator to your abstract Event class as follows:static Random generator = new Random(1);//use a seed of 1 Note that we have seeded the random number generator with 1. This is to ensure that we will always generate the same output each time we run the code. This will help you to test your code more easily and will also allow the markers to check your work for this version of the program.
Your concrete event classes must now use this random number generator to:
1. Determine how long after an arrival before the OrderEvent is to be scheduled (values to be between 1 and 5 time units).
2. Determine the number of people in a group (to be between 1 and 4 people in a group).
3. Determine how long after getting their coffee the group stayed in the shop, i.e. how long after the OrderEvent before the LeaveEvent is to be scheduled to occur (values to be between 5 and 12 time units).
Questions
Regardless of which phase you have completed, Include the answers to the following questions in your report.
Question 1: Why does the random number generator in phase 6 need to be static?
Question 2: What is the default access modifier for an instance variable if we don’t specify an access modifier and what does it mean in terms of what other classes can access the instance variable?
Question 3: How could you restrict the instance variables in Event to only the subclasses of Event?
Solution
Instructions
A description of what is required in each section is shown in italics. Please remove all the instructions from your final report.
Description of the Program and Phase Completed
Phase 1 has been completed
In this phase we have define the all classes given in the class diagram.
Phase 2 has been completed
Added serveOrder method to ShopModal class and defined ServeEvent so that customer can get coffee served by stipulated time interval.
Phase 3 has been completed
In this phase we have define leave method in to shop modal class and defined a new LeaveEvent class to track the customer group leaving time
Phase 4 has been completed
We have fixed the total number of seats available at the shop in the beginning so that after arriving of each customer group we can see whether the customer group will got seat or leave the coffee house with out placing order
Phase 5 has been completed
Define the working of statistics file so that simulator can writhe the details of program as per given in the format.
Phase 6 has been completed
Created the random class instance into event class so that the automatic random number will be generated and tested the program accordingly.
Testing
Testing of scheduling of events
Testing of group of people in each customer group
Sample Output
Simulation trace to the standard output:
Statistics written to the statistics.txt file
Bugs and Limitations
Bugs
“No known bugs”
Limitations
I don’t think any limitations
Additional Future Work
We can add multithreading to simulate the same project
Answers to Questions
Question 1
The event class can use directly because we are unable to create object of abstract class.
Question 2
The default access modifier of any reference variable of class is called default. That reference variable can be access by anywhere within the same package with the help of object.
Question 3
We can use private access modifier so that it can be used in subclass but not with the help of object in anywhere of the program.
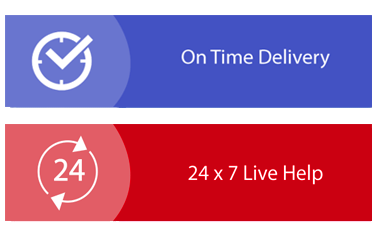
Download Samples PDF
Related Sample
- SPO101 Introduction To Sports Management Assignment
- Is AI Taking Over the Work of Financial Analysts
- ACCT6005 Company Accounting
- BSB40215 Diploma Certificate IV in Business
- TCHR5003 Principles and Practices in Early Childhood Assignment
- MBA642 Project Initiation, Planning and Execution Report 3
- BE955 International Marketing and Entrepreneurship Assignment
- BDA601 Big Data and Analytics Report
- Marketing Fundamental Report
- LAW2442 Commercial Law Assignment
- MGT601 Dynamic Leadership Report
- PUBH6013 Qualitative Research Methods Report
- ENEM28001 FEA for Engineering Design Report
- Internet Use and Economic Development Evidence and Policy Implications Assignment
- MIS501 Principles of Programming Learning Activity Assignment
- MGT602 Business Decision Analytics Research Report 3
- BSBHRM525 Manage Recruitment and Onboarding Assignment
- MBA673 Business Analytics Lifecycle Report 3
- BIS3003 IS Capstone Industry Project A Assignment
- BUACC5930 Accounting Concepts and Practice Essay
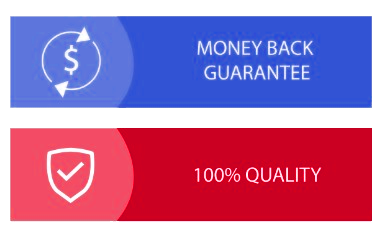
Assignment Services
-
Assignment Writing
-
Academic Writing Services
- Urgent Assignment Help
- Writing Assignment for University
- College Assignment Help
- SPSS Assignment Help
- HND Assignment Help
- Architecture Assignment Help
- Total Assignment Help
- All Assignment Help
- My Assignment Help
- Student Assignment Help
- Instant Assignment Help
- Cheap Assignment Help
- Global Assignment Help
- Write My Assignment
- Do My Assignment
- Solve My Assignment
- Make My Assignment
- Pay for Assignment Help
-
Management
- Financial Management Assignment Help
- Business Management Assignment Help
- Management Assignment Help
- Project Management Assignment Help
- Supply Chain Management Assignment Help
- Operations Management Assignment Help
- Risk Management Assignment Help
- Strategic Management Assignment Help
- Logistics Management Assignment Help
- Global Business Strategy Assignment Help
- Consumer Behavior Assignment Help
- MBA Assignment Help
- Portfolio Management Assignment Help
- Change Management Assignment Help
- Hospitality Management Assignment Help
- Healthcare Management Assignment Help
- Investment Management Assignment Help
- Market Analysis Assignment Help
- Corporate Strategy Assignment Help
- Conflict Management Assignment Help
- Marketing Management Assignment Help
- Strategic Marketing Assignment Help
- CRM Assignment Help
- Marketing Research Assignment Help
- Human Resource Assignment Help
- Business Assignment Help
- Business Development Assignment Help
- Business Statistics Assignment Help
- Business Ethics Assignment Help
- 4p of Marketing Assignment Help
- Pricing Strategy Assignment Help
- Nursing
-
Finance
- Finance Assignment Help
- Do My Finance Assignment For Me
- Financial Accounting Assignment Help
- Behavioral Finance Assignment Help
- Finance Planning Assignment Help
- Personal Finance Assignment Help
- Financial Services Assignment Help
- Forex Assignment Help
- Financial Statement Analysis Assignment Help
- Capital Budgeting Assignment Help
- Financial Reporting Assignment Help
- International Finance Assignment Help
- Business Finance Assignment Help
- Corporate Finance Assignment Help
-
Accounting
- Accounting Assignment Help
- Managerial Accounting Assignment Help
- Taxation Accounting Assignment Help
- Perdisco Assignment Help
- Solve My Accounting Paper
- Business Accounting Assignment Help
- Cost Accounting Assignment Help
- Taxation Assignment Help
- Activity Based Accounting Assignment Help
- Tax Accounting Assignment Help
- Financial Accounting Theory Assignment Help
-
Computer Science and IT
- Robotics Assignment Help
- Operating System Assignment Help
- Data mining Assignment Help
- Computer Network Assignment Help
- Database Assignment Help
- IT Management Assignment Help
- Network Topology Assignment Help
- Data Structure Assignment Help
- Business Intelligence Assignment Help
- Data Flow Diagram Assignment Help
- UML Diagram Assignment Help
- R Studio Assignment Help
-
Law
- Law Assignment Help
- Business Law Assignment Help
- Contract Law Assignment Help
- Tort Law Assignment Help
- Social Media Law Assignment Help
- Criminal Law Assignment Help
- Employment Law Assignment Help
- Taxation Law Assignment Help
- Commercial Law Assignment Help
- Constitutional Law Assignment Help
- Corporate Governance Law Assignment Help
- Environmental Law Assignment Help
- Criminology Assignment Help
- Company Law Assignment Help
- Human Rights Law Assignment Help
- Evidence Law Assignment Help
- Administrative Law Assignment Help
- Enterprise Law Assignment Help
- Migration Law Assignment Help
- Communication Law Assignment Help
- Law and Ethics Assignment Help
- Consumer Law Assignment Help
- Science
- Biology
- Engineering
-
Humanities
- Humanities Assignment Help
- Sociology Assignment Help
- Philosophy Assignment Help
- English Assignment Help
- Geography Assignment Help
- Agroecology Assignment Help
- Psychology Assignment Help
- Social Science Assignment Help
- Public Relations Assignment Help
- Political Science Assignment Help
- Mass Communication Assignment Help
- History Assignment Help
- Cookery Assignment Help
- Auditing
- Mathematics
-
Economics
- Economics Assignment Help
- Managerial Economics Assignment Help
- Econometrics Assignment Help
- Microeconomics Assignment Help
- Business Economics Assignment Help
- Marketing Plan Assignment Help
- Demand Supply Assignment Help
- Comparative Analysis Assignment Help
- Health Economics Assignment Help
- Macroeconomics Assignment Help
- Political Economics Assignment Help
- International Economics Assignments Help
-
Academic Writing Services
-
Essay Writing
- Essay Help
- Essay Writing Help
- Essay Help Online
- Online Custom Essay Help
- Descriptive Essay Help
- Help With MBA Essays
- Essay Writing Service
- Essay Writer For Australia
- Essay Outline Help
- illustration Essay Help
- Response Essay Writing Help
- Professional Essay Writers
- Custom Essay Help
- English Essay Writing Help
- Essay Homework Help
- Literature Essay Help
- Scholarship Essay Help
- Research Essay Help
- History Essay Help
- MBA Essay Help
- Plagiarism Free Essays
- Writing Essay Papers
- Write My Essay Help
- Need Help Writing Essay
- Help Writing Scholarship Essay
- Help Writing a Narrative Essay
- Best Essay Writing Service Canada
-
Dissertation
- Biology Dissertation Help
- Academic Dissertation Help
- Nursing Dissertation Help
- Dissertation Help Online
- MATLAB Dissertation Help
- Doctoral Dissertation Help
- Geography Dissertation Help
- Architecture Dissertation Help
- Statistics Dissertation Help
- Sociology Dissertation Help
- English Dissertation Help
- Law Dissertation Help
- Dissertation Proofreading Services
- Cheap Dissertation Help
- Dissertation Writing Help
- Marketing Dissertation Help
- Programming
-
Case Study
- Write Case Study For Me
- Business Law Case Study Help
- Civil Law Case Study Help
- Marketing Case Study Help
- Nursing Case Study Help
- Case Study Writing Services
- History Case Study help
- Amazon Case Study Help
- Apple Case Study Help
- Case Study Assignment Help
- ZARA Case Study Assignment Help
- IKEA Case Study Assignment Help
- Zappos Case Study Assignment Help
- Tesla Case Study Assignment Help
- Flipkart Case Study Assignment Help
- Contract Law Case Study Assignments Help
- Business Ethics Case Study Assignment Help
- Nike SWOT Analysis Case Study Assignment Help
- Coursework
- Thesis Writing
- CDR
- Research