Order Now
- Home
- About Us
-
Services
-
Assignment Writing
-
Academic Writing Services
- Urgent Assignment Help
- Writing Assignment for University
- College Assignment Help
- SPSS Assignment Help
- HND Assignment Help
- Architecture Assignment Help
- Total Assignment Help
- All Assignment Help
- My Assignment Help
- Student Assignment Help
- Instant Assignment Help
- Cheap Assignment Help
- Global Assignment Help
- Write My Assignment
- Do My Assignment
- Solve My Assignment
- Make My Assignment
- Pay for Assignment Help
-
Management
- Financial Management Assignment Help
- Business Management Assignment Help
- Management Assignment Help
- Project Management Assignment Help
- Supply Chain Management Assignment Help
- Operations Management Assignment Help
- Risk Management Assignment Help
- Strategic Management Assignment Help
- Logistics Management Assignment Help
- Global Business Strategy Assignment Help
- Consumer Behavior Assignment Help
- MBA Assignment Help
- Portfolio Management Assignment Help
- Change Management Assignment Help
- Hospitality Management Assignment Help
- Healthcare Management Assignment Help
- Investment Management Assignment Help
- Market Analysis Assignment Help
- Corporate Strategy Assignment Help
- Conflict Management Assignment Help
- Marketing Management Assignment Help
- Strategic Marketing Assignment Help
- CRM Assignment Help
- Marketing Research Assignment Help
- Human Resource Assignment Help
- Business Assignment Help
- Business Development Assignment Help
- Business Statistics Assignment Help
- Business Ethics Assignment Help
- 4p of Marketing Assignment Help
- Pricing Strategy Assignment Help
- Nursing
-
Finance
- Finance Assignment Help
- Do My Finance Assignment For Me
- Financial Accounting Assignment Help
- Behavioral Finance Assignment Help
- Finance Planning Assignment Help
- Personal Finance Assignment Help
- Financial Services Assignment Help
- Forex Assignment Help
- Financial Statement Analysis Assignment Help
- Capital Budgeting Assignment Help
- Financial Reporting Assignment Help
- International Finance Assignment Help
- Business Finance Assignment Help
- Corporate Finance Assignment Help
-
Accounting
- Accounting Assignment Help
- Managerial Accounting Assignment Help
- Taxation Accounting Assignment Help
- Perdisco Assignment Help
- Solve My Accounting Paper
- Business Accounting Assignment Help
- Cost Accounting Assignment Help
- Taxation Assignment Help
- Activity Based Accounting Assignment Help
- Tax Accounting Assignment Help
- Financial Accounting Theory Assignment Help
-
Computer Science and IT
- Robotics Assignment Help
- Operating System Assignment Help
- Data mining Assignment Help
- Computer Network Assignment Help
- Database Assignment Help
- IT Management Assignment Help
- Network Topology Assignment Help
- Data Structure Assignment Help
- Business Intelligence Assignment Help
- Data Flow Diagram Assignment Help
- UML Diagram Assignment Help
- R Studio Assignment Help
-
Law
- Law Assignment Help
- Business Law Assignment Help
- Contract Law Assignment Help
- Tort Law Assignment Help
- Social Media Law Assignment Help
- Criminal Law Assignment Help
- Employment Law Assignment Help
- Taxation Law Assignment Help
- Commercial Law Assignment Help
- Constitutional Law Assignment Help
- Corporate Governance Law Assignment Help
- Environmental Law Assignment Help
- Criminology Assignment Help
- Company Law Assignment Help
- Human Rights Law Assignment Help
- Evidence Law Assignment Help
- Administrative Law Assignment Help
- Enterprise Law Assignment Help
- Migration Law Assignment Help
- Communication Law Assignment Help
- Law and Ethics Assignment Help
- Consumer Law Assignment Help
- Science
- Biology
- Engineering
-
Humanities
- Humanities Assignment Help
- Sociology Assignment Help
- Philosophy Assignment Help
- English Assignment Help
- Geography Assignment Help
- Agroecology Assignment Help
- Psychology Assignment Help
- Social Science Assignment Help
- Public Relations Assignment Help
- Political Science Assignment Help
- Mass Communication Assignment Help
- History Assignment Help
- Cookery Assignment Help
- Auditing
- Mathematics
-
Economics
- Economics Assignment Help
- Managerial Economics Assignment Help
- Econometrics Assignment Help
- Microeconomics Assignment Help
- Business Economics Assignment Help
- Marketing Plan Assignment Help
- Demand Supply Assignment Help
- Comparative Analysis Assignment Help
- Health Economics Assignment Help
- Macroeconomics Assignment Help
- Political Economics Assignment Help
- International Economics Assignments Help
-
Academic Writing Services
-
Essay Writing
- Essay Help
- Essay Writing Help
- Essay Help Online
- Online Custom Essay Help
- Descriptive Essay Help
- Help With MBA Essays
- Essay Writing Service
- Essay Writer For Australia
- Essay Outline Help
- illustration Essay Help
- Response Essay Writing Help
- Professional Essay Writers
- Custom Essay Help
- English Essay Writing Help
- Essay Homework Help
- Literature Essay Help
- Scholarship Essay Help
- Research Essay Help
- History Essay Help
- MBA Essay Help
- Plagiarism Free Essays
- Writing Essay Papers
- Write My Essay Help
- Need Help Writing Essay
- Help Writing Scholarship Essay
- Help Writing a Narrative Essay
- Best Essay Writing Service Canada
-
Dissertation
- Biology Dissertation Help
- Academic Dissertation Help
- Nursing Dissertation Help
- Dissertation Help Online
- MATLAB Dissertation Help
- Doctoral Dissertation Help
- Geography Dissertation Help
- Architecture Dissertation Help
- Statistics Dissertation Help
- Sociology Dissertation Help
- English Dissertation Help
- Law Dissertation Help
- Dissertation Proofreading Services
- Cheap Dissertation Help
- Dissertation Writing Help
- Marketing Dissertation Help
- Programming
-
Case Study
- Write Case Study For Me
- Business Law Case Study Help
- Civil Law Case Study Help
- Marketing Case Study Help
- Nursing Case Study Help
- Case Study Writing Services
- History Case Study help
- Amazon Case Study Help
- Apple Case Study Help
- Case Study Assignment Help
- ZARA Case Study Assignment Help
- IKEA Case Study Assignment Help
- Zappos Case Study Assignment Help
- Tesla Case Study Assignment Help
- Flipkart Case Study Assignment Help
- Contract Law Case Study Assignments Help
- Business Ethics Case Study Assignment Help
- Nike SWOT Analysis Case Study Assignment Help
- Coursework
- Thesis Writing
- CDR
- Research
-
Assignment Writing
-
Resources
- Referencing Guidelines
-
Universities
-
Australia
- Asia Pacific International College Assignment Help
- Macquarie University Assignment Help
- Rhodes College Assignment Help
- APIC University Assignment Help
- Torrens University Assignment Help
- Kaplan University Assignment Help
- Holmes University Assignment Help
- Griffith University Assignment Help
- VIT University Assignment Help
- CQ University Assignment Help
-
Australia
- Experts
- Free Sample
- Testimonial
BIS1003 Introduction To Programming Assignment Sample
Assessment 4 Details:
EduWra Company pointed you to develop a program to assist in predicting the customer's preferences. This company sells books online. The program would calculate the average rating for all the books in the dataset and display the highest rated books. We could better predict what a customer might like by considering the customer's actual ratings in the past and how these ratings compare to the ratings given by other customers.
The assessment has two tasks – Task 1: implement the program in Python. Task 2: write a formal report.
Task 1:
Implement the program in Python. Comment on your code as necessary to explain it clearly. Your task for this project is to write a program that takes people's book ratings and makes book recommendations to others using the average rating technique. The program will read customers data from a text file (you find the text file on Canvas site for this unit) that contains sets of three lines about each customer. The first line will have the customer's username, the second line book title, and the third line the rating that is given to the book.
Task 2:
Each group should write a formal report that includes:
- A cove page for your assignment contains the group members' names and contribution percentages (each student must state which parts of the project have been completed). If your name is not on the cover page, you will be given zero.
- Draw system flowchart/s that present the steps of the algorithm required to perform the major system tasks.
- You need to test your program for assignment help by selecting at least three sets of testing data. Provide the results of testing your program with different values and record the testing results using the testing table below.
- Copy the code to your report.
- Take screenshots of the program output.
Solution
Flowchart
1. Read ratings.txt file
2. Store each bookname from given books
3. Get Rating for each user and corresponding books
4. Get recommendation
Source Code
# Assignment 4 : Applied Project
# Name : Jenil, Rohan, Mayank_group_11
# date : 12/12/2021
# Group No: 11
#this function read all the books information and return
def readBooksName(books):
i = 1
bookNames = []
while True:
if i > len(books):
break
# if bookNames is not founc in the list then only add the bookname
if bookNames.count(books[i].strip()) == 0:
#remove the new line or extra spaces from bookname
bookNames.append(books[i].strip())
#increase the line by 3 to get next book
i = i + 3
return bookNames
#this function will find the rating of each book and store into dictionary
def getRating(books, bookNames):
ratings = {} #create empty dictionary
i = 0
while True:
if i > len(books) - 1: #break loop if reached to last record
break
ratings[books[i].strip()] = [0] * len(bookNames) #create list of zero for each book
i = i + 3 #increase counter by three to read next user name
i = 0
while True:
if i > len(books) - 1:
break
key = books[i].strip()
bookName = books[i+1].strip()
try:
rating = int(books[i+2])
except ValueError:
print('Error: Book rating must be int')
rating = 0
index = bookNames.index(bookName)
#update rating for paricular index book
ratings[key][index] = rating
#increase couter by 3
i = i + 3
return ratings
def calcAverageRating(bookRatings, bookNames):
#create empty list to store avgRatings
avgRatings = []
#get all the ratings of books
values = list(bookRatings.values())
for i in range(len(bookNames)):
sum = 0
count = 0
for ratings in values:
#get the correspoding rating value from values list
sum += ratings[i]
if ratings[i] != 0: #count only not zero value
count += 1
avgRatings.append(sum/count) #calculating avg rating and append to the list
#sorting the avg rating and corresponding bookNames
for i in range(len(avgRatings)):
for j in range(len(avgRatings)):
if avgRatings[i] > avgRatings[j]:
avgRatings[i], avgRatings[j] = avgRatings[j],avgRatings[i]
bookNames[i],bookNames[j] = bookNames[j], bookNames[i]
return avgRatings,bookNames
def showAvgRatings(bookNames,bookAvgRatings ):
for i in range(len(bookNames)):
print('The average ratings for %s is %.2f' % (bookNames[i], bookAvgRatings[i]))
def showRecommendation(bookList):
for book in bookList:
if book[1] != 0:
print('The average ratings for %s is %.2f' % (book[0], book[1]))
def findRecommendation(customerName,bookRatings,bookNames):
givenUser = bookRatings[customerName]
recomendations = []
#retrieve each books and rating values for each user
for key, value in bookRatings.items():
if key != customerName: #take only customer other than given customername
similarity = [] #create empty list to store multiplication of each user
for i in range(len(value)):
similarity.append(value[i] * givenUser[i]) #store the multiplication value
recomendations.append((key, sum(similarity))) #store the sum of similarity value
recomendations.sort(key=lambda x: x[1]) #sorting them by rating
recomendations.reverse() #reverse to get topThree
topThree = recomendations[:3] #getting topthree customer with highest similiarity
bookList = [] #empty list to store booklist
recommend = [0] * len(bookNames) #creating empty list to stroe recommend
for i in range(len(bookNames)):
s = 0
c = 0
for j in topThree:
s = s + bookRatings[j[0]][i] #getting rating value of each user for partiuclar book
if bookRatings[j[0]][i] != 0: #count only for non zero value
c += 1
if c != 0: #find out avg rating where count is non zero
recommend[i] = s / c
bookList.append((bookNames[i], recommend[i])) #append bookname and avg rating to booklist
bookList.sort(key=lambda x: x[1]) #sorting the booklist by rating value
bookList.reverse() #reverse the booklist to get in decending order
return bookList
def main():
try:
fp = open('data3.txt', 'r') #if file not found return with error message
books = fp.readlines()
except IOError:
print('Error: File not found')
return
bookNames = readBooksName(books)
bookRatings = getRating(books, bookNames)
bookAvgRatings, bookNames = calcAverageRating(bookRatings,bookNames)
while True:
print('\n\nWelcome to the EduWra Book Recommendation System.')
print('1: All books average ratings')
print('2: recommend books for a particular user')
print('q: exit the program')
choice = input('\nSelect one of the options above: ')
if choice == 'q':
break
elif choice == '1':
showAvgRatings(bookNames,bookAvgRatings)
elif choice == '2':
customerName = input('\nPlease enter customer name: ')
if customerName in bookRatings:
bookList = findRecommendation(customerName, bookRatings,bookNames)
showRecommendation(bookList)
else:
showAvgRatings(bookNames, bookAvgRatings)
if __name__ == '__main__':
main()
Test Data Table 1
Output details of Test Data 1
Test Data Table 2
Test Data Table 3
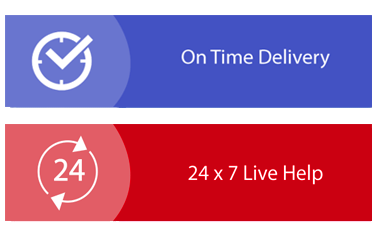
Download Samples PDF
Related Sample
- PUBH6013 Qualitative Research Methods 3A Report
- ECO600 Economics and Finance for Business Assignment
- MBA642 Project Initiation Planning and Execution
- MIS500 Foundations of Information Systems Report 3
- BSBITU404 Produce Complex Desktop Published Document Assignment
- EDUC1001 Language and Learning in Your Discipline Assignment
- MCR003 Management Attributes and Skills Assignment
- HI5016 International Trade and Enterprise Assignment
- BRH606 Business Research for Hoteliers
- BE553 Principles of International Marketing Assignment
- Dynamic Leadership Assignment
- BSBSUS511 Develop Workplace Policy and Procedure Assignment
- ISYS1004 Contemporary issue in Information Technology Assignment
- 7069SOH Global Healthcare Management Report
- MIS501 Principles of Programming Learning Activity Assignment
- PSYC1003 Understanding Mind Brain and Behaviour Assignment
- PRJ5106 Research Methodology and Data Analysis Report 1
- CSE2HUM Ethical Issue on Current Cyber Context Assignment
- ITECH7410 Software Engineering Methodologies Assignment
- LMED28001 Chemical Pathology Case Study 1
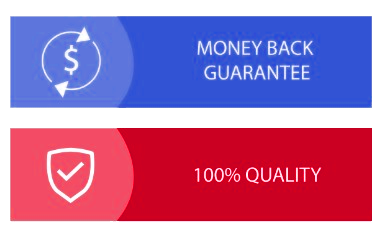
Assignment Services
-
Assignment Writing
-
Academic Writing Services
- Urgent Assignment Help
- Writing Assignment for University
- College Assignment Help
- SPSS Assignment Help
- HND Assignment Help
- Architecture Assignment Help
- Total Assignment Help
- All Assignment Help
- My Assignment Help
- Student Assignment Help
- Instant Assignment Help
- Cheap Assignment Help
- Global Assignment Help
- Write My Assignment
- Do My Assignment
- Solve My Assignment
- Make My Assignment
- Pay for Assignment Help
-
Management
- Financial Management Assignment Help
- Business Management Assignment Help
- Management Assignment Help
- Project Management Assignment Help
- Supply Chain Management Assignment Help
- Operations Management Assignment Help
- Risk Management Assignment Help
- Strategic Management Assignment Help
- Logistics Management Assignment Help
- Global Business Strategy Assignment Help
- Consumer Behavior Assignment Help
- MBA Assignment Help
- Portfolio Management Assignment Help
- Change Management Assignment Help
- Hospitality Management Assignment Help
- Healthcare Management Assignment Help
- Investment Management Assignment Help
- Market Analysis Assignment Help
- Corporate Strategy Assignment Help
- Conflict Management Assignment Help
- Marketing Management Assignment Help
- Strategic Marketing Assignment Help
- CRM Assignment Help
- Marketing Research Assignment Help
- Human Resource Assignment Help
- Business Assignment Help
- Business Development Assignment Help
- Business Statistics Assignment Help
- Business Ethics Assignment Help
- 4p of Marketing Assignment Help
- Pricing Strategy Assignment Help
- Nursing
-
Finance
- Finance Assignment Help
- Do My Finance Assignment For Me
- Financial Accounting Assignment Help
- Behavioral Finance Assignment Help
- Finance Planning Assignment Help
- Personal Finance Assignment Help
- Financial Services Assignment Help
- Forex Assignment Help
- Financial Statement Analysis Assignment Help
- Capital Budgeting Assignment Help
- Financial Reporting Assignment Help
- International Finance Assignment Help
- Business Finance Assignment Help
- Corporate Finance Assignment Help
-
Accounting
- Accounting Assignment Help
- Managerial Accounting Assignment Help
- Taxation Accounting Assignment Help
- Perdisco Assignment Help
- Solve My Accounting Paper
- Business Accounting Assignment Help
- Cost Accounting Assignment Help
- Taxation Assignment Help
- Activity Based Accounting Assignment Help
- Tax Accounting Assignment Help
- Financial Accounting Theory Assignment Help
-
Computer Science and IT
- Robotics Assignment Help
- Operating System Assignment Help
- Data mining Assignment Help
- Computer Network Assignment Help
- Database Assignment Help
- IT Management Assignment Help
- Network Topology Assignment Help
- Data Structure Assignment Help
- Business Intelligence Assignment Help
- Data Flow Diagram Assignment Help
- UML Diagram Assignment Help
- R Studio Assignment Help
-
Law
- Law Assignment Help
- Business Law Assignment Help
- Contract Law Assignment Help
- Tort Law Assignment Help
- Social Media Law Assignment Help
- Criminal Law Assignment Help
- Employment Law Assignment Help
- Taxation Law Assignment Help
- Commercial Law Assignment Help
- Constitutional Law Assignment Help
- Corporate Governance Law Assignment Help
- Environmental Law Assignment Help
- Criminology Assignment Help
- Company Law Assignment Help
- Human Rights Law Assignment Help
- Evidence Law Assignment Help
- Administrative Law Assignment Help
- Enterprise Law Assignment Help
- Migration Law Assignment Help
- Communication Law Assignment Help
- Law and Ethics Assignment Help
- Consumer Law Assignment Help
- Science
- Biology
- Engineering
-
Humanities
- Humanities Assignment Help
- Sociology Assignment Help
- Philosophy Assignment Help
- English Assignment Help
- Geography Assignment Help
- Agroecology Assignment Help
- Psychology Assignment Help
- Social Science Assignment Help
- Public Relations Assignment Help
- Political Science Assignment Help
- Mass Communication Assignment Help
- History Assignment Help
- Cookery Assignment Help
- Auditing
- Mathematics
-
Economics
- Economics Assignment Help
- Managerial Economics Assignment Help
- Econometrics Assignment Help
- Microeconomics Assignment Help
- Business Economics Assignment Help
- Marketing Plan Assignment Help
- Demand Supply Assignment Help
- Comparative Analysis Assignment Help
- Health Economics Assignment Help
- Macroeconomics Assignment Help
- Political Economics Assignment Help
- International Economics Assignments Help
-
Academic Writing Services
-
Essay Writing
- Essay Help
- Essay Writing Help
- Essay Help Online
- Online Custom Essay Help
- Descriptive Essay Help
- Help With MBA Essays
- Essay Writing Service
- Essay Writer For Australia
- Essay Outline Help
- illustration Essay Help
- Response Essay Writing Help
- Professional Essay Writers
- Custom Essay Help
- English Essay Writing Help
- Essay Homework Help
- Literature Essay Help
- Scholarship Essay Help
- Research Essay Help
- History Essay Help
- MBA Essay Help
- Plagiarism Free Essays
- Writing Essay Papers
- Write My Essay Help
- Need Help Writing Essay
- Help Writing Scholarship Essay
- Help Writing a Narrative Essay
- Best Essay Writing Service Canada
-
Dissertation
- Biology Dissertation Help
- Academic Dissertation Help
- Nursing Dissertation Help
- Dissertation Help Online
- MATLAB Dissertation Help
- Doctoral Dissertation Help
- Geography Dissertation Help
- Architecture Dissertation Help
- Statistics Dissertation Help
- Sociology Dissertation Help
- English Dissertation Help
- Law Dissertation Help
- Dissertation Proofreading Services
- Cheap Dissertation Help
- Dissertation Writing Help
- Marketing Dissertation Help
- Programming
-
Case Study
- Write Case Study For Me
- Business Law Case Study Help
- Civil Law Case Study Help
- Marketing Case Study Help
- Nursing Case Study Help
- Case Study Writing Services
- History Case Study help
- Amazon Case Study Help
- Apple Case Study Help
- Case Study Assignment Help
- ZARA Case Study Assignment Help
- IKEA Case Study Assignment Help
- Zappos Case Study Assignment Help
- Tesla Case Study Assignment Help
- Flipkart Case Study Assignment Help
- Contract Law Case Study Assignments Help
- Business Ethics Case Study Assignment Help
- Nike SWOT Analysis Case Study Assignment Help
- Coursework
- Thesis Writing
- CDR
- Research